hibernate连接各种数据库(mysql、ms sql、Oracle)
用户库:
mysql
mysql-connector-java-3.1.13-bin.jar
mssql
sqljdbc4.jar
oracle10g
ojdbc14_g.jar
配置文件
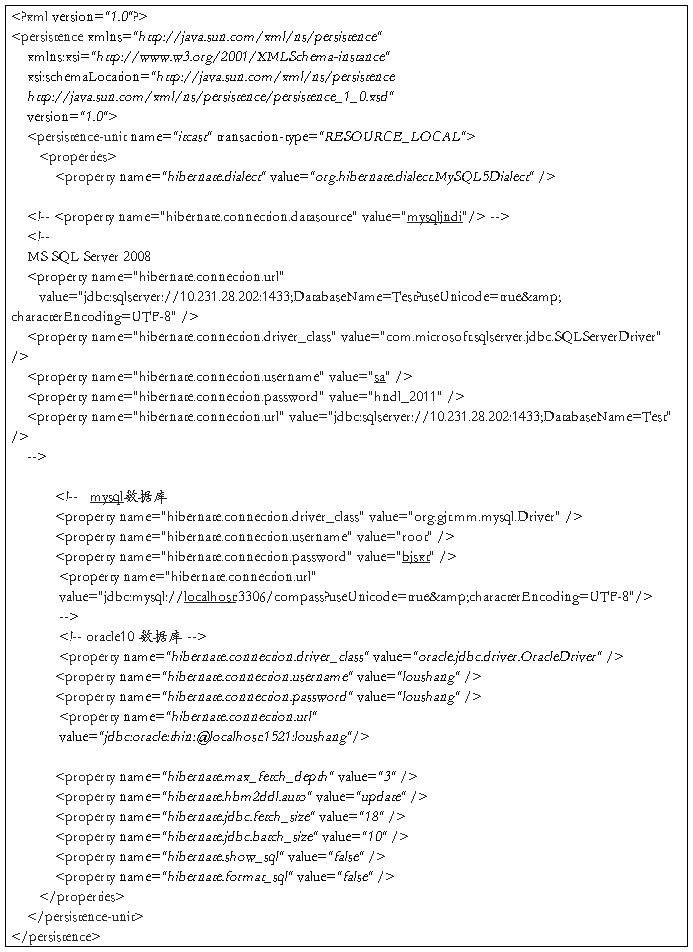
工具准备:
需要包:
hibernate-annotation-3.4.0.GA.zip
hibernate-distribution-3.3.2.GA-dist.zip
slf4j-1.5.8.zip
mysql-connector-java-3.1.13-bin.jar
-----------------------------------------------------------------
hibernate-annotation-3.4.0.GA.zip
hibernate-annotations.jar
\lib\ejb3-persistence.jar
\lib\hibernate-commons-annoations.jar
---------------------------------------------
hibernate-distribution-3.3.2.GA-dist.zip
hibernate3.jar
lib\required\antlr-2.7.6.jar
lib\required\commons-collections-3.1.jar
lib\required\dom4j-1.6.1.jar
lib\required\javassist-3.9.GA.jar
lib\required\jta-1.1.jar
lib\required\slf4j-api-1.5.8.jar
--------------------------------
slf4j-1.5.8.zip
slf4j-nop-1.5.8.jar
打开eclipse,Windows->Preferences->User Libraries
添加hibernate、hibernateAnnotation、slf4j、mysql四个用户库,分别导入jar包
第一个hibernate例子(*.hbm.xml)
创建Java Project工程,hibernate,将四个用户库导入,
目录结构如下图所示:
源代码
hibernate.cfg.xml内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/hibernate?useUnicode=true&characterEncoding=UTF-8</property>
<property name="connection.username">root</property>
<property name="connection.password">bjsxt</property>
<property name="connection.pool">1</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">update</property>
<mapping resource="Person.hbm.xml"/>
<!-- <mapping class="com.hibernate.bean.Person" /> -->
</session-factory>
</hibernate-configuration>
Person.java内容如下:
package com.hibernate.bean;
publicclass Person {
private String id;
private String name;
public Person() {
}
public Person(String id, String name) {
this.id = id;
this.name = name;
}
public String getId() {
return id;
}
publicvoid setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
publicvoid setName(String name) {
this.name = name;
}
}
Person.hbm.xml内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd" >
<hibernate-mapping package="com.hibernate.bean">
<class name="Person" table="person">
<id name="id" column="id" type="string">
</id>
<property name="name" column="name" type="string" ></property>
</class>
</hibernate-mapping>
PersonTest.java内容如下:
package junit.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import org.junit.BeforeClass;
import org.junit.Test;
import com.hibernate.bean.Person;
publicclass PersonTest {
privatestatic Session session;
@BeforeClass
publicstaticvoid setUpBeforeClass() throws Exception {
Configuration cfg=new Configuration();
SessionFactory sessionFactory=cfg.configure().buildSessionFactory();
session=sessionFactory.openSession();
}
@Test
publicvoid testSavePerson() {
try {
session.beginTransaction();
session.save(new Person("sno1", "zhangsan"));
session.getTransaction().commit();
} catch (Exception e) {
e.printStackTrace();
session.getTransaction().rollback();
} finally {
if (session != null && !session.isOpen()) {
session.close();
}
}
}
@Test
publicvoid testQuery(){
Person person=(Person)session.get(Person.class, "sno1");
System.out.println(person.getId()+person.getName());
}
}
Annotation(@Entity)例子
hibernate.cfg.xml:内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/hibernate?useUnicode=true&characterEncoding=UTF-8</property>
<property name="connection.username">root</property>
<property name="connection.password">bjsxt</property>
<property name="connection.pool">1</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">update</property>
<!-- <mapping resource="Person.hbm.xml"/>-->
<mapping class="com.hibernate.bean.Person" />
</session-factory>
</hibernate-configuration>
Person.java内容如下:
package com.hibernate.bean;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
publicclass Person {
private String id;
private String name;
public Person() {
}
public Person(String id, String name) {
this.id = id;
this.name = name;
}
@Id
@Column(length=20)
public String getId() {
return id;
}
publicvoid setId(String id) {
this.id = id;
}
@Column(length=20)
public String getName() {
return name;
}
publicvoid setName(String name) {
this.name = name;
}
}
PersonTest.java内容如下:
package junit.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.AnnotationConfiguration;
import org.hibernate.cfg.Configuration;
import org.junit.BeforeClass;
import org.junit.Test;
import com.hibernate.bean.Person;
publicclass PersonTest {
privatestatic Session session;
@BeforeClass
publicstaticvoid setUpBeforeClass() throws Exception {
Configuration cfg=new AnnotationConfiguration();
SessionFactory sessionFactory=cfg.configure().buildSessionFactory();
session=sessionFactory.openSession();
}
@Test
publicvoid testSavePerson() {
try {
session.beginTransaction();
session.save(new Person("sno1", "zhangsan"));
session.getTransaction().commit();
} catch (Exception e) {
e.printStackTrace();
session.getTransaction().rollback();
} finally {
if (session != null && !session.isOpen()) {
session.close();
}
}
}
@Test
publicvoid testQuery(){
Person person=(Person)session.get(Person.class, "sno1");
System.out.println(person.getId()+person.getName());
}
}
Spring+hibernate
程序目录如下:
用户库:
hibernate用户库
hibernate-distribution-3.3.2.GA\hibernate3.jar
hibernate-distribution-3.3.2.GA\lib\required\antlr-2.7.6.jar
hibernate-distribution-3.3.2.GA\lib\required\commons-collections-3.1.jar
hibernate-distribution-3.3.2.GA\lib\required\dom4j-1.6.1.jar
hibernate-distribution-3.3.2.GA\lib\required\javassist-3.9.0.GA.jar
hibernate-distribution-3.3.2.GA\lib\required\jta-1.1.jar
hibernate-distribution-3.3.2.GA\lib\required\slf4j-api-1.5.8.jar
hibernateAnnotation用户库
hibernate-annotations-3.4.0.GA\hibernate-annotations.jar
hibernate-annotations-3.4.0.GA\lib\hibernate-commons-annotations.jar
hibernate-annotations-3.4.0.GA\lib\ejb3-persistence.jar
mysql用户库
mysql-connector-java-3.1.13-bin.jar
spring2.5.6用户库
spring-framework-2.5.6\dist\spring.jar
spring-framework-2.5.6\lib\jakarta-commons\commons-logging.jar
spring-framework-2.5.6\lib\jakarta-commons\commons-pool.jar
spring-framework-2.5.6\lib\jakarta-commons\commons-dbcp.jar
spring-framework-2.5.6\lib\j2ee\common-annotations.jar
spring-framework-2.5.6\lib\aspectj\aspectjrt.jar
spring-framework-2.5.6\lib\aspectj\aspectjweaver.jar
spring-framework-2.5.6\lib\cglib\cglib-nodep-2.1_3.jar
slf4j用户库
slf4j-1.5.8\slf4j-nop-1.5.8.jar
源代码
Person.java
package com.hibernate.bean;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Person {
private String personId;
private String name;
public Person() {
}
public Person(String personId, String name) {
this.personId = personId;
this.name = name;
}
@Id
@Column(length=20)
public String getPersonId() {
return personId;
}
public void setPersonId(String personId) {
this.personId = personId;
}
@Column(length=20)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
IPersonService.java
package com.hibernate.service;
import java.util.List;
import com.hibernate.bean.Person;
public interface IPersonService {
public void save(Person person);
public void delete(String personid);
public void update(Person person);
public Person getPerson(String personid);
public List<Person> getPersonList();
}
PersonServiceBean.java
package com.hibernate.service.impl;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.orm.hibernate3.HibernateTemplate;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.hibernate.bean.Person;
import com.hibernate.service.IPersonService;
@Service
@Transactional
publicclass PersonServiceBean implements IPersonService {
@Resource
private HibernateTemplate hibernateTemplate;
publicvoid save(Person person){
hibernateTemplate.save(person);
}
publicvoid delete(String personid){
hibernateTemplate.delete(hibernateTemplate.get(Person.class, personid));
}
publicvoid update(Person person){
hibernateTemplate.merge(person);
}
public Person getPerson(String personid){
return (Person)hibernateTemplate.get(Person.class, personid);
}
public List<Person> getPersonList(){
returnhibernateTemplate.find("from Person");
}
}
beans.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
<context:component-scan base-package="com.hibernate" />
<bean id="sessionFactory"
class="org.springframework.orm.hibernate3.annotation.AnnotationSessionFactoryBean">
<property name="packagesToScan" value="com.hibernate.*" />
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLInnoDBDialect</prop>
<prop key="hibernate.connection.driver_class">com.mysql.jdbc.Driver</prop>
<prop key="hibernate.connection.url">jdbc:mysql://localhost:3306/compass?useUnicode=true&characterEncoding=UTF-8
</prop>
<prop key="hibernate.connection.username">root</prop>
<prop key="hibernate.connection.password">bjsxt</prop>
<prop key="hibernate.hbm2ddl.auto">update</prop>
<prop key="hibernate.format_sql">false</prop>
<prop key="hibernate.show_sql">false</prop>
</props>
</property>
</bean>
<bean id="hibernateTemplate" class="org.springframework.orm.hibernate3.HibernateTemplate">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
<bean id="txManager"
class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
<aop:config>
<aop:pointcut id="bussinessService"
expression="execution(public * com.hibernate.service.*.*(..))" />
<aop:advisor pointcut-ref="bussinessService" advice-ref="txAdvice" />
</aop:config>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="*" propagation="REQUIRED" />
</tx:attributes>
</tx:advice>
<!---->
<!-- 等同于上面AOP拦截配置 -->
<!-- <tx:annotation-driven transaction-manager="txManager" /> -->
</beans>
PersonTest.java
package junit.test;
import java.util.List;
import org.junit.BeforeClass;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.hibernate.bean.Person;
import com.hibernate.service.IPersonService;
publicclass PersonTest {
privatestatic IPersonService personService;
@BeforeClass
publicstaticvoid setUpBeforeClass() throws Exception {
ApplicationContext applicationContext=new ClassPathXmlApplicationContext("beans.xml");
personService=(IPersonService)applicationContext.getBean("personServiceBean");
}
@Test
publicvoid testSave() {
personService.save(new Person("sno4","张三"));
}
@Test
publicvoid testUpdate(){
personService.save(new Person("sno3","李四"));
}
@Test
publicvoid testQueryList(){
List<Person> persons=personService.getPersonList();
for(Person person: persons){
System.out.println(person.getPersonId()+person.getName());
}
}
@Test
publicvoid testDelete(){
personService.delete("sno2");
}
@Test
publicvoid testQuery(){
Person person=personService.getPerson("sno3");
System.out.println(person.getPersonId()+person.getName());
}
}
Spring+hibernate+JPA
目录结构如下图
所需组件:
hibernate-annotations-3.4.0.GA.zip
hibernate-distribution-3.3.2.GA-dist.zip
hibernate-entitymanager-3.3.2.GA.zip
mysql-connector-java-3.1.13-bin.jar
slf4j-1.5.8.zip
用户库
所需jar包明细
hibernate-annotations-3.4.0.GA.zip
hibernate-annotations-3.4.0.GA\hibernate-annotations.jar
hibernate-annotations-3.4.0.GA\lib\hibernate-commons-annotations.jar
hibernate-annotations-3.4.0.GA\lib\ejb3-persistence.jar
hibernate-distribution-3.3.2.GA-dist.zip
hibernate-distribution-3.3.2.GA\hibernate3.jar
hibernate-distribution-3.3.2.GA\lib\required\antlr-2.7.6.jar
hibernate-distribution-3.3.2.GA\lib\required\commons-collections-3.1.jar
hibernate-distribution-3.3.2.GA\lib\required\dom4j-1.6.1.jar
hibernate-distribution-3.3.2.GA\lib\required\javassist-3.9.0.GA.jar
hibernate-distribution-3.3.2.GA\lib\required\jta-1.1.jar
hibernate-distribution-3.3.2.GA\lib\required\slf4j-api-1.5.8.jar
hibernate-entitymanager-3.3.2.GA.zip
hibernate-entitymanager-3.3.2.GA\hibernate-entitymanager.jar
slf4j-1.5.8.zip
slf4j-1.5.8\slf4j-nop-1.5.8.jar
项目实例:
Person.java
package com.hibernate.bean;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Person {
private String id;
private String name;
public Person() {
}
public Person(String id, String name) {
this.id = id;
this.name = name;
}
@Id
@Column(length = 20)
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
@Column(length = 20)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
IPersonService.java
package com.hibernate.service;
import java.util.List;
import com.hibernate.bean.Person;
publicinterface IPersonService {
publicvoid save(Person person);
publicvoid update(Person person);
publicvoid delete(Person person);
public Person getPerson(String personid);
public List<Person> getPersonList();
}
PersonServiceBean.java
package com.hibernate.service.impl;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.hibernate.bean.Person;
import com.hibernate.service.IPersonService;
@Transactional
@Service
publicclass PersonServiceBean implements IPersonService {
@PersistenceContext
private EntityManager em;
publicvoid save(Person person){
em.persist(person);
}
publicvoid update(Person person){
em.merge(person);
}
publicvoid delete(String personid){
em.remove(em.getReference(Person.class, personid));
}
public Person getPerson(String personid){
return em.find(Person.class, personid);
}
public List<Person> getPersonList(){
returnem.createQuery("from Person ").getResultList();
}
}
persistence.xml
<?xml version="1.0"?>
<persistence xmlns="http://java.sun.com/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_1_0.xsd"
version="1.0">
<persistence-unit name="itcast" transaction-type="RESOURCE_LOCAL">
<properties>
<property name="hibernate.dialect" value="org.hibernate.dialect.MySQL5Dialect" />
<!-- <property name="hibernate.connection.datasource" value="mysqljndi"/>
<property name="hibernate.connection.url" value="jdbc:sqlserver://10.231.28.202:1433;DatabaseName=Test?useUnicode=true&characterEncoding=UTF-8"/>
<property name="hibernate.connection.driver_class" value="com.microsoft.sqlserver.jdbc.SQLServerDriver"/>
<property name="hibernate.connection.username" value="sa"/> <property name="hibernate.connection.password"
value="hndl_2011"/> <property name="hibernate.connection.url" value="jdbc:sqlserver://10.231.28.202:1433;DatabaseName=Test"/>
<property name="hibernate.max_fetch_depth" value="3"/> <property name="hibernate.hbm2ddl.auto"
value="update"/> <property name="hibernate.show_sql" value="false"/> <property
name="hibernate.format_sql" value="false"/> -->
<!-- -->
<property name="hibernate.connection.driver_class" value="org.gjt.mm.mysql.Driver" />
<property name="hibernate.connection.username" value="root" />
<property name="hibernate.connection.password" value="bjsxt" />
<!-- <property name="hibernate.connection.url" value="jdbc:mysql://localhost:3306/qingfeng?useUnicode=true&characterEncoding=UTF-8"/> -->
<property name="hibernate.connection.url"
value="jdbc:mysql://localhost:3306/compass?useUnicode=true&characterEncoding=gbk" />
<property name="hibernate.max_fetch_depth" value="3" />
<property name="hibernate.hbm2ddl.auto" value="update" />
<property name="hibernate.show_sql" value="false" />
<property name="hibernate.format_sql" value="false" />
</properties>
</persistence-unit>
</persistence>
beans.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
<context:component-scan base-package="com.hibernate"/>
<context:annotation-config />
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalEntityManagerFactoryBean">
<property name="persistenceUnitName" value="itcast"/>
</bean>
<!-- -->
<bean id="txManager" class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory" ref="entityManagerFactory"/>
</bean>
<tx:annotation-driven transaction-manager="txManager"/>
</beans>
PersonTest.java
package junit.test;
import java.util.List;
import org.junit.BeforeClass;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.hibernate.bean.Person;
import com.hibernate.service.IPersonService;
public class PersonTest {
private static IPersonService personService;
@BeforeClass
public static void setUpBeforeClass() throws Exception {
ApplicationContext applicationContext=new ClassPathXmlApplicationContext("beans.xml");
personService=(IPersonService)applicationContext.getBean("personServiceBean");
}
@Test
public void testSave() {
Person person=new Person("sno1","张三");
personService.save(person);
}
@Test
public void testUpdate() {
Person person=new Person("sno1","李四");
personService.update(person);
}
@Test
public void testDelete() {
personService.delete("sno1");
}
@Test
public void testGetPerson() {
Person person=personService.getPerson("sno1");
System.out.println("标识: "+person.getId()+" 姓名:"+person.getName());
}
@Test
public void testGetPersonList() {
List<Person> persons=personService.getPersonList();
for(Person person:persons){
System.out.println("标识: "+person.getId()+" 姓名:"+person.getName());
}
}
}
常见问题答疑
Caused by: javax.persistence.PersistenceException: No Persistence provider for EntityManager named itcast
hibernate版本不一样,配置文件的DTD声明不同