温湿度计校验记录
文件编码:
校验日期: 年 月 日
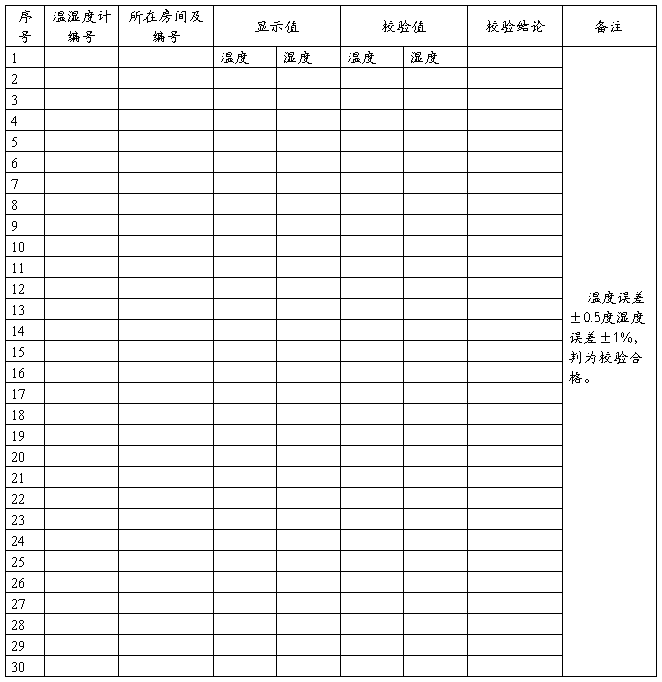
校验人: 复核人:
第二篇:温湿度计程序
/***********************************************************************************
Project: SHTxx 驱动程序 4位数码管移位扫描显示
***********************************************************************************/
#include <reg51.h> //Microcontroller specific library, e.g. port definitions
#include <intrins.h> //Keil library (is used for _nop()_ operation)
#define uchar unsigned char
#define uint unsigned int
#define outdata P0 //8951mcu
enum {TEMP,HUMI};
sbit DATA=P2^1;
sbit SCK=P2^0;
#define noACK 0
#define ACK 1
#define MEASURE_TEMP 0x03 //000 0001 1
#define MEASURE_HUMI 0x05 //000 0010 1
#define RESET 0x1e //000 1111 0
//-------------------硬件配置----------------------------------
//P0口为段码数据,P1.4, P1.5, P1.6, P0.5 分别为1234位数码管
//===================显示段码数据==============================
uchar duanma[10]={0x28,0xeb,0x32,0xa2,0xe1,0xa4,0x24,0xea,0x20,0xa0};
//===================位控制===================================
sbit WEI1=P2^7;
sbit WEI2=P2^6;
sbit WEI3=P2^5;
sbit WEI4=P2^4; //8951
//====================按键配置=================================
//==========================显示缓冲区RAM配置==================
uchar buffer[4]={0,0,0,0};
uchar wd,sd;
union
{ unsigned int i;
float f;
}wendu,shidu;//定义两个共同体,一个用于湿度,一个用于温度
//====================================================
void delayHM(uint k)//毫秒级延时子函数
{
uint i;
uchar j;
for(i=0;i<k;i++)
{
for(j=0;j<121;j++);
}
}
//=================移位扫描显示函数===================== void led_dispy(uchar bu[])
{
uchar i;
for(i=0;i<4;i++)
{
outdata=duanma[bu[i]];
switch(i)//位选控制
{
case 0: WEI1=0;break;
case 1: WEI2=0;break;
case 2: WEI3=0;break;
case 3: WEI4=0;break;
}
delayHM(1);
WEI1=WEI2=WEI3=WEI4=1;
}
}
char s_write_byte(unsigned char value)
//---------------------------------------------------------------------------------- // 写字节函数
{
unsigned char i,error=0;
for (i=0x80;i>0;i/=2) //shift bit for masking
{ if (i & value) DATA=1; //masking value with i , write to SENSI-BUS else DATA=0;
SCK=1; //clk for SENSI-BUS
_nop_();_nop_();_nop_(); //pulswith approx. 5 us
SCK=0;
}
DATA=1; //release DATA-line
SCK=1; //clk #9 for ack
error=DATA; //check ack (DATA will be pulled down by SHT11) SCK=0;
return error; //error=1 in case of no acknowledge
}
//---------------------------------------------------------------------------------- char s_read_byte(unsigned char ack)
//---------------------------------------------------------------------------------- //读数据;
{
unsigned char i,val=0;
DATA=1; //release DATA-line
for (i=0x80;i>0;i/=2) //shift bit for masking
{ SCK=1; //clk for SENSI-BUS
if (DATA) val=(val | i); //read bit
SCK=0;
}
DATA=!ack; //in case of "ack==1" pull down DATA-Line SCK=1; //clk #9 for ack
_nop_();_nop_();_nop_(); //pulswith approx. 5 us
SCK=0;
DATA=1; //release DATA-line
return val;
}
//---------------------------------------------------------------------------------- void s_transstart(void)
//---------------------------------------------------------------------------------- // generates a transmission start
// _____ ________
// DATA: |_______|
// ___ ___
// SCK : ___| |___| |______
{
DATA=1; SCK=0; //Initial state
_nop_();
SCK=1;
_nop_();
DATA=0;
_nop_();
SCK=0;
_nop_();
SCK=1;
_nop_();
DATA=1;
_nop_();
SCK=0;
}
//----------------------------------------------------------------------------------
void s_connectionreset(void)
//----------------------------------------------------------------------------------
// //启动传输
// _____________________________________________________ ________
// DATA: |_______|
// _ _ _ _ _ _ _ _ _ ___ ___
// SCK : __| |__| |__| |__| |__| |__| |__| |__| |__| |______| |___| |______
{
unsigned char i;
DATA=1; SCK=0; //Initial state
for(i=0;i<9;i++) //9 SCK cycles
{ SCK=1;
SCK=0;
}
s_transstart();
}
//----------------------------------------------------------------------------------
char s_measure(unsigned char *p_value, unsigned char *p_checksum, unsigned char mode) //----------------------------------------------------------------------------------
// 进行温度或者湿度转换,由参数mode决定转换内容;
{
unsigned error=0;
unsigned int i;
s_transstart(); //transmission start
switch(mode){ //send command to sensor
case TEMP : error+=s_write_byte(MEASURE_TEMP); break;
case HUMI : error+=s_write_byte(MEASURE_HUMI); break;
default : break;
}
for (i=0;i<600;i++){ led_dispy(buffer);if(DATA==0) break;} //wait until sensor has finished the measurement
if(DATA) error+=1; // or timeout (~2 sec.) is reached
*(p_value) =s_read_byte(ACK); //read the first byte (MSB)
*(p_value+1)=s_read_byte(ACK); //read the second byte (LSB)
*p_checksum =s_read_byte(noACK); //read checksum
return error;
}
//----------------------------------------------------------------------------------
//----------------------------------------------------------------------------------------
void calc_sth11(float *p_humidity ,float *p_temperature)
//----------------------------------------------------------------------------------------
// 补偿及输出温度和相对湿度
// input : humi [Ticks] (12 bit)
// temp [Ticks] (14 bit)
// output: humi [%RH]
// temp [度]
{ const float C1=-4.0; // for 12 Bit
const float C2=+0.0405; // for 12 Bit
const float C3=-0.0000028; // for 12 Bit
const float T1=+0.01; // for 14 Bit @ 5V
const float T2=+0.00008; // for 14 Bit @ 5V
float rh=*p_humidity; // rh: Humidity [Ticks] 12 Bit
float t=*p_temperature; // t: Temperature [Ticks] 14 Bit
float rh_lin; // rh_lin: Humidity linear
float rh_true; // rh_true: Temperature compensated humidity
float t_C; // t_C : Temperature
t_C=t*0.01 - 40; //calc. temperature from ticks
rh_lin=C3*rh*rh + C2*rh + C1; //calc. humidity from ticks to [%RH]
rh_true=(t_C-25)*(T1+T2*rh)+rh_lin; //calc. temperature compensated humidity [%RH] if(rh_true>100)rh_true=100; //cut if the value is outside of
if(rh_true<0.1)rh_true=0.1; //the physical possible range
*p_temperature=t_C; //return temperature
*p_humidity=rh_true; //return humidity[%RH]
}
//--------------------------------------------------------------------
void main()
//--------------------------------------------------------------------
{
uchar error,checksum;
uint xunh;
s_connectionreset();
while(1)
{
xunh++;
if(xunh==4000){xunh=0;
error=0;
error+=s_measure((unsigned char*) &shidu.i,&checksum,HUMI); //湿度测量 error+=s_measure((unsigned char*) &wendu.i,&checksum,TEMP); //温度测量 if(error!=0) s_connectionreset(); //如果发生错误,系统复位 else
{ shidu.f=(float)shidu.i; //转换为浮点数
wendu.f=(float)wendu.i; //转换为浮点数
calc_sth11(&shidu.f,&wendu.f); //修正相对湿度及温度
sd=(char)shidu.f;
wd=(char)wendu.f;
buffer[0]=sd%10;
buffer[1]=sd/10;
buffer[2]=wd%10;
buffer[3]=wd/10;
led_dispy(buffer);
}
}
//-------------------------------------------------------
led_dispy(buffer);
}
}